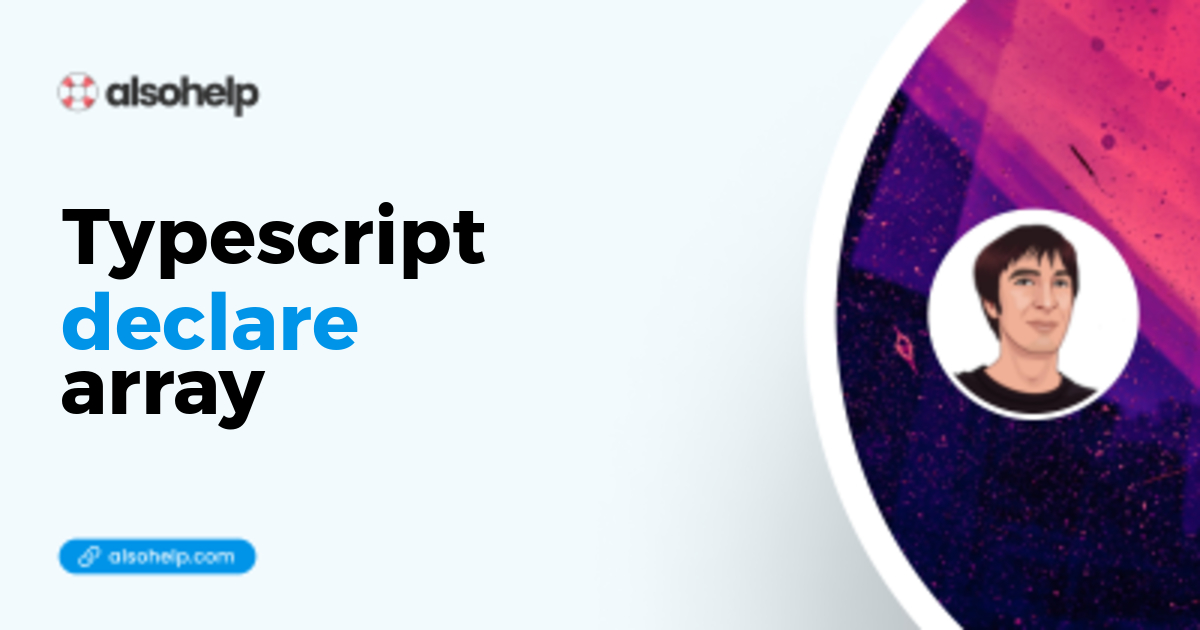
· typescript · 1 min read
Declare an array in TypeScript
How to declare an array in TypeScript the right way. A small recap, mostly note to self.
First, this is how you declare an array in JS :
In JS
const fruits = ['apple', 'orange', 'banana'];
Remember that TS generates JS, so you always end up with the above.
In TS
In TS, it will look like this:
const fruits: string[] = ['apple', 'orange', 'banana'];
You are telling TS “I’m declaring an array of string”.
In TS, you always declare an array of something.
- an Array of string,
- an Array of number,
- an Array of MyType,
and so on.
Things to avoid
There is no “array of nothing” in TS.
❌ const fruits: [] = []
// wrong! you are declaring an empty array
❌ const fruits = [1, 2, 3]
// wrong! you are declaring an "array of any", like in JS
❌ const fruits: any[] = [1, 2, 3]
// wrong! you are using any, which is almost forbidden in TS
Summary
An array in TS is always an “array of something”. I think it’s the only gotcha to remember.
Best,
David